Functional Programming & Functional JavaScript: A Beginner's Guide
Functional programming is a powerful tool for developing efficient and maintainable codebases. By leveraging the benefits of this paradigm, developers can create highly performant applications that are easy to read, understand, and extend.

Functional programming is a powerful tool for developing efficient and maintainable codebases. By leveraging the benefits of this paradigm, developers can create highly performant applications that are easy to read, understand, and extend.
In this blog post, we will explore what functional programming is and discuss its advantages over other paradigms. We'll take an in-depth look at key concepts such as pure functions, composition, declarative style coding and conciseness.
Additionally, we'll provide examples of how functional programming can be used for data manipulation or mathematical operations and explain why it is ideal for these types of problems.
Finally, we will discuss the advantages of using a functional approach when writing code. So let's get started!
- Functional programming is a powerful tool for creating efficient and maintainable codebases.
- Leverage the benefits of functional programming to create highly performant applications that are easy to read, understand, and extend.
- Learn about key concepts such as pure functions, composition, declarative style coding and conciseness.
- Understand how functional programming can be used for data manipulation or mathematical operations and why it is ideal for these tasks.
- Discover the advantages of using a functional approach when writing code.
What is functional programming?
Functional programming is a powerful approach to software development that encourages the use of reusable, modular codebases.
This paradigm focuses on functions instead of variables, encouraging effective composition and abstraction. Developers can create highly performant applications that are easier to read, maintain, and extend.
At its core, functional programming emphasizes the use of pure functions. These are functions that take in input and return a result without modifying any external state. This allows developers to create applications with predictable behaviour, reducing the risk of errors due to unexpected changes within the system.
Additionally, by avoiding mutation of variables, it is much easier for developers to reason about and debug their code.
Functional programming also advocates for a declarative style of coding, which seeks to describe what the program should do without specifying how it should be done. This allows developers to focus on their intent, instead of their implementation details. Additionally, by using concise functions and composition techniques, they can create succinct code that is easy to understand and maintain.
Finally, functional programming is ideal for tasks that involve data manipulation or mathematical operations. By taking advantage of functions such as map, filter and reduce, developers can quickly and easily perform complex calculations with just a few lines of code. This makes it much easier to work with large datasets efficiently and accurately.
Functional programming provides developers with a powerful and flexible approach to writing code. By using this paradigm, they can create maintainable applications that are easier to read and debug. Additionally, it is ideal for problems involving data manipulation or mathematical operations due to its concise style and composition techniques. Therefore, functional programming can be an invaluable tool for any developer looking to create highly efficient codebases.
- Functional programming allows developers to create highly performant applications with a minimum of code.
- Use pure functions to create predictable, reliable behaviour and reduce debugging time.
- Declarative style coding focuses on intent instead of implementation details for easier readability.
- Leverage composition techniques to write concise code that is easy to understand and maintain.
- Utilize powerful data manipulation tools such as map, filter and reduce for efficient mathematical operations on large datasets.
- Take advantage of the benefits of functional programming in order to achieve maximum efficiency when building software.

The Art of Functional Programming
Functional programming is a powerful and elegant programming paradigm. It allows for highly concise solutions with increased safety. This has led to rising demand for software engineers with functional programming skills. This book will help you move your programming skills to the next level.
What are the benefits of functional programming?
Functional programming is a powerful programming paradigm that enables developers to create efficient and maintainable code. It is based on the idea of pure functions, which are functions that return the same output for a given input and do not produce any side effects. This makes it much easier to debug and modify code as needed.
Furthermore, functional programming emphasizes a declarative style of coding that focuses on intent instead of implementation details. This makes it much easier to read and understand code, leading to better maintainability. Additionally, by leveraging composition techniques such as map, filter and reduce, developers can write concise code that is easier to extend when changes are needed.
Finally, functional programming is great for tasks such as data manipulation or mathematical operations. By taking advantage of these powerful tools, developers can quickly and accurately perform calculations on large datasets without having to write long sections of code.
Overall, the advantages of functional programming are clear: it leads to more efficient and maintainable code that is easier to read, debug and extend. For these reasons, functional programming is quickly becoming the language of choice for many developers.
- Functional programming reduces side effects, making code more reliable and easier to debug.
- By emphasizing intent over implementation, developers can write better maintainable code.
- Composition techniques such as map, filter and reduce make it easier to generate complex applications with shorter codebases.
- Functional programming is ideal for data manipulation or mathematical operations that require quick and accurate calculations on large datasets.
- The advantages of functional programming include efficient code that is easy to read and maintain, fewer side effects and improved reliability.
What are the core concepts of functional programming?
Functional programming is a powerful paradigm that allows developers to create efficient and maintainable codebases. It relies on key concepts such as functions, values, and variables which help to break down complex problems into simpler pieces. In this section, we will discuss the core concepts of functional programming in detail and look at how they can be used to create reliable applications.
- Functions are the fundamental building blocks of functional programming.
- Values in functional programming represent actual data instead of variables or constants.
- Variables are temporary storage for information while a program is running, and values can change over time as the function progresses.
- Functional programming encourages composition, which is the process of combining two or more functions to create a new one.
- Finally, functional programming encourages the use of pure functions with no side effects.
1. Functions
Functions are the fundamental building blocks of functional programming. They take one or more arguments (values) and return a value based on those parameters. Functions enable us to simplify complex problems by breaking them down into smaller pieces that can be solved independently.
2. Values
Values in functional programming represent actual data instead of variables or constants. Variables are temporary storage for information while a program is running, and values can change over time as the function progresses.
3. Variables
Variables are used to store values that can then be operated on by functions. Variables should not be mutated within a function, as this will lead to errors and unintended behavior.
4. Composition
Functional programming encourages composition, which is the process of combining two or more functions to create a new one. This makes it possible to create powerful abstractions that can be used for data manipulation or mathematical operations.
5. Declarative Style
By combining functions, we can also create code that is more concise and declarative in style, which makes it easier to read and maintain.
6. Pure Functions
Finally, functional programming encourages the use of pure functions with no side effects. This means that the same result is always returned for a given set of parameters, making it possible to write extremely testable and bug-free code.
7. Viewing Programs as Collections of Functions
Functional programming encourages developers to view their applications as a collection of functions, instead of classes or objects. This allows for simpler and more maintainable codebases that are easier to debug and extend.

Grokking Simplicity: Taming complex software with functional thinking
Distributed across servers, difficult to test, and resistant to modification—modern software is complex. Grokking Simplicity is a friendly, practical guide that will change the way you approach software design and development.
It introduces a unique approach to functional programming that explains why certain features of software are prone to complexity, and teaches you the functional techniques you can use to simplify these systems so that they’re easier to test and debug.
What is a pure function?
A pure function is a function that does not modify its arguments, and always produces the same output for a given set of inputs. This is achieved by not having any side effects or relying on external state.
Pure functions are a key concept in functional programming, as they make it easier to reason about code and create maintainable and testable applications. They can be used for calculations or data manipulation, as they guarantee the same result every time they are called. Additionally, pure functions can be easily tested by simply calling them and checking that they produce the expected results without having to worry about anything else going on behind the scenes.
Finally, pure functions can be composed together to create powerful abstractions that make code easier to understand and maintain.
What is a higher-order function?
A higher-order function is a special type of function that takes one or more functions as arguments and returns a new function as the result of applying the given functions. Higher-order functions are powerful tools in functional programming, allowing developers to quickly create complex operations by combining simpler ones.
This can be helpful when writing programs that need to handle multiple tasks at once, as they make it easier to keep code organized and efficient. Additionally, these types of functions enable developers to write more efficient code by reducing the amount of duplicate code within an application.
What is a closure?
A closure is a function that can be assigned to a variable.
Closures are functions that can be stored in variables. When you define a closure, you're telling the compiler that this function takes two arguments: the current context and some object. The context is an object that contains information about the environment in which the closure was executed (for example, how many arguments were passed in). The object also allows you to access global variables and properties of objects within the current scope.
When you assign a closure to a variable, it becomes available for use within the same scope as where it was defined. This means that closures are extremely versatile tools for organizing your code and making sure related code is accessible from one place.
Closures can also be used to create more complex functions by combining simpler closures together into larger ones.

You Don't Know JS Yet: Scope & Closures
The foundation of all programs is the organization of its variables and functions into different nested scopes. Yet, most developers haven't deeply contemplated how and why these decisions are made and the impacts on code maintainability.
Scope & Closures examines all aspects of lexical scope, then builds on these principles to leverage the power of closure, and finally digs into the module pattern for better program structure.
What is currying?
Currying is a functional programming technique that allows you to modularize your code by transforming one function into another.
Currying is a functional programming technique that lets you transform one function into another. This means that you can break your code up into smaller, more manageable pieces, and it'll be easier to reuse this code in the future.
This method of coding makes it possible to handle multiple functions at once without having to write separate functions for each one. Instead, you can simply call the original function with the first parameter as the second parameter.
For example, say you have a function called adder that takes two numbers and adds them together:
adder(1, 2) returns 3; adder(3, 4) returns 5; // etc...
You could also rewrite this same code using currying:
adder = (a, b) => a + b; // etc...
In this case, we're defining an anonymous function (an unnamed piece of code), which takes two parameters (a and b). We then define an instance of this anonymous function by passing in 1 and 2 as input parameters. Next we call our anonymousfunction with 3 as its first parameter and 4 as its secondparameter, and we get the result 5.
What is memoization?
Memoization is a technique that helps you save memory by caching results of a function call.
Memoization can help you speed up your code by caching the results of a function call. This means that instead of calling the function multiple times, the computer will only need to lookup the result once.
This can save lots of memory and make your code run faster.
Memoization is especially useful when you're working with complex functions that take a long time to calculate their result.
What is recursion?
Recursion is a type of programming that involves repeating a certain task or procedure over and over again.
Recursion can be used to solve problems in programming by repeating a certain task or procedure until it's complete.
In recursive programming, the program calls itself repeatedly with the new data that's been collected.
This process can be repeated as many times as necessary to solve the problem.
Recursive code is usually more concise than non-recursive code because it doesn't need to keep track of all the details of the previous execution step.
Recursive code is easier to read and understand because you can see how each part of the program works together.
What are some of the drawbacks of functional programming?
Some of the drawbacks of functional programming include that it can be difficult to write code that is concise and easy to read. Additionally, functional programming may not be well-suited for certain tasks, such as data manipulation or machine learning.
Is JavaScript truly a functional language?
JavaScript is a functional language, but it's not truly functional because it has some features that are not typical of Functional Programming.
Functional programming is a style of programming that focuses on writing code that can be executed without any errors. This makes the code more reliable and easier to read.
JavaScript does have some features that make it closer to being a true functional language, but there are still some areas in which it falls short.
Some of the main differences between JavaScript and Functional Programming include: variables can be assigned once and never changed again; functions can return multiple values; and functions can take arguments (which are passed into the function as strings).

Mastering JavaScript Functional Programming, 2nd Edition
Write clean, robust, and maintainable web and server code using functional JavaScript. Explore the functional programming paradigm and the different techniques for developing better algorithms, writing more concise code, and performing seamless testing.
TypeScript and functional programming
TypeScript is a language that allows developers to use functional programming. It uses the JavaScript code as its foundation and is created by Microsoft.
TypeScript offers many benefits from using functional programming such as improved code readability and maintainability, better tooling support from IDEs (integrated development environments), a faster compilation time when using TypeScript's ECMAScript 6 features.
TypeScript offers great support for functional programming and provides some of the key concepts such as pure functions, composition, declarative style and conciseness. This makes it ideal for developing applications with complex logic or operations that involve large amounts of data manipulation.
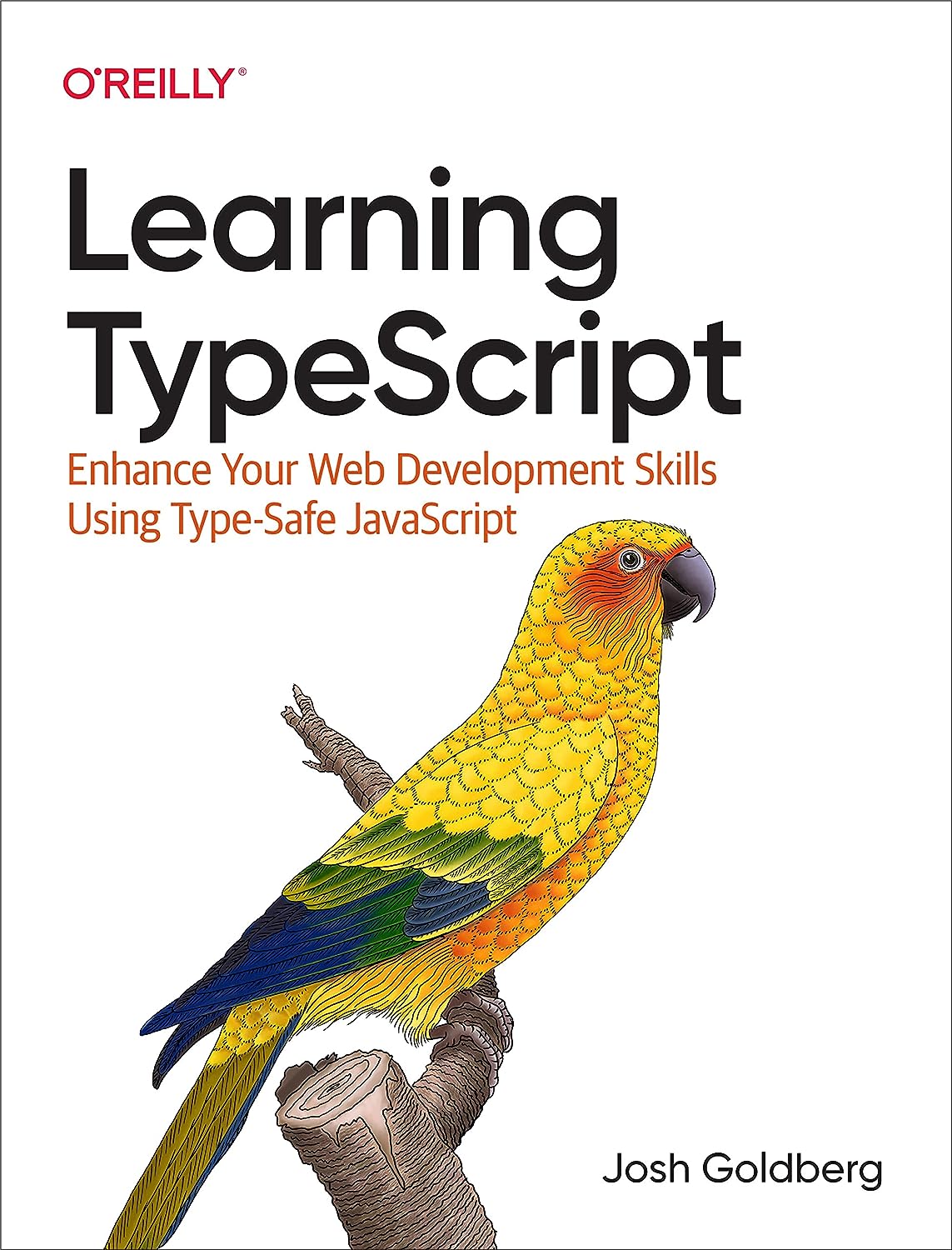
Learning TypeScript
TypeScript has conquered the world of JavaScript. It's widely used across the world and is frequently credited for helping massive web applications scale.
This practical book takes beginner and advanced JavaScript programmers alike from knowing nothing about "types" or "type systems" to full mastery of TypeScript fundamentals.
Conclusion
In conclusion, Functional Programming is an important paradigm when it comes to coding. It offers many benefits such as improved readability, maintainability, and faster compilation times. It can be used for data manipulation or mathematical operations with great efficiency and is ideal for these types of problems.
Finally, when using TypeScript it offers even more advantages such as better tooling support from IDEs and the ability to use ECMAScript 6 features. So if you are looking for a way to make your code read cleaner and run faster, then Functional Programming is definitely worth exploring.