How to Make HTTP Requests with JavaScript
Learn how to make HTTP requests with JavaScript, exploring the different methods available such as XMLHttpRequest, Fetch, and Axios. Learn best practices for making HTTP requests via JavaScript, and the considerations to be taken when doing so.
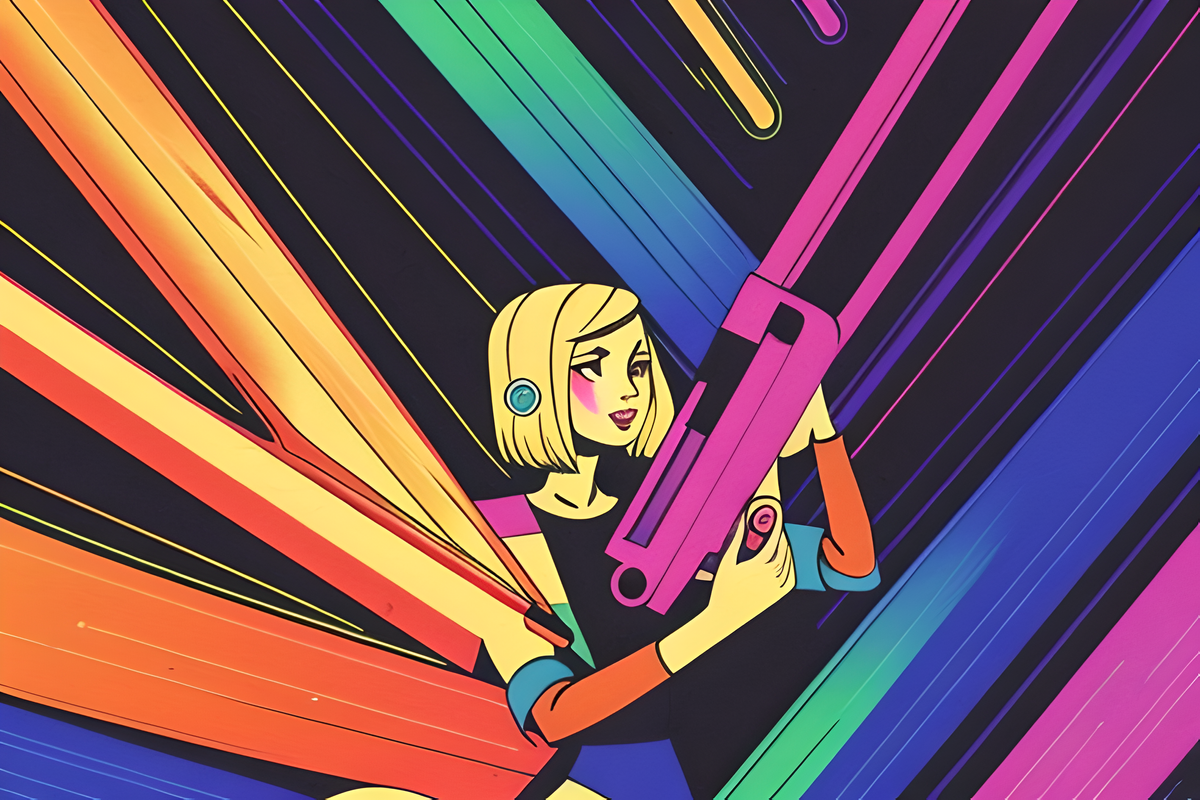
Make your website more efficient by learning how to make HTTP requests with JavaScript. The most popular way to do this is via the XMLHttpRequest object, which can be used to fetch data from web servers and exchange information between clients and servers.
We'll also explore Fetch and other libraries like Axios for making HTTP requests, as well as best practices and considerations when making requests. Get up to speed quickly and maximize your website's performance with this comprehensive guide on making HTTP requests in JavaScript!
How do I make an HTTP request in Javascript?
There are several ways to make HTTP requests in JavaScript. The most common way is to use the XMLHttpRequest
object, which encapsulates all the necessary methods and properties for making an HTTP request. The XMLHttpRequest
object can be used to make both asynchronous and synchronous requests.
Another popular method of making HTTP requests in JavaScript is by using the Fetch API. Fetch is a modern replacement for XMLHttpRequest
and provides an easy-to-use interface for making requests. It also supports more features than XMLHttpRequest
, such as streaming responses, automatic JSON parsing and more.
In addition to these two methods, there are a number of third-party libraries available that provide an even easier way to make HTTP requests in JavaScript. These libraries provide a simple API for making requests, and often include features such as automatic JSON parsing and other useful utilities.
Use XMLHttpRequest
One way is to use the XMLHttpRequest
object, which is supported by modern web browsers.
Here is an example of how to use the XMLHttpRequest
object to make an HTTP GET
request to a server:
// Create a new XMLHttpRequest object
const xhr = new XMLHttpRequest();
// Open a connection to the server
xhr.open('GET', 'https://example.com/api/v1/data', true);
// Set the request headers
xhr.setRequestHeader('Content-Type', 'application/json');
// Send the request
xhr.send();
// Handle the response
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
The XMLHttpRequest
object has a number of properties and methods that can be used to make HTTP requests. The open()
method is used to open a connection to the server, and the send()
method is used to send the request to the server.
The setRequestHeader()
method is used to set the request headers, and the onreadystatechange()
method is used to handle the response from the server. The readyState
property is used to determine the state of the request, and the status
property is used to determine the status of the response.
The above code snippet is an example of how to make an HTTP GET
request to a server. The same approach can be used to make other types of requests, such as POST
, PUT
, DELETE
, and so on.
Use Fetch
Another way to make HTTP requests in JavaScript is to use the fetch()
function, which is supported by modern web browsers. It is easier to use than XMLHttpRequest
and has more capabilities. Here is an example of how to use the fetch()
function to make an HTTP GET request:
// Make a GET request to the server
fetch('https://example.com/api/v1/data')
// Parse the response as JSON
.then(response => response.json())
// Log the response to the console
.then(data => console.log(data))
// Handle any errors
.catch(error => console.log(error));
The fetch()
function is used to make an HTTP request to a server. It takes a single argument, which is the URL of the server. The fetch()
function returns a Promise that resolves to a Response object.
The json()
method is used to parse the response as JSON, and the catch()
method is used to handle any errors that occur.
Fetch Response object methods & properties
The Response object has a number of methods and properties that can be used to handle the response from the server.
response.json()
is used to parse the response as JSON.response.text()
is used to parse the response as text.response.ok
is used to determine if the response was successful.response.status
is used to determine the status of the response.response.statusText
is used to determine the status text of the response.response.headers
is used to get the response headers.response.url
is used to get the URL of the response.
Use a library like Axios
You can also use libraries like Axios or Superagent to make HTTP requests in JavaScript. These libraries provide a simpler interface for making HTTP requests and can be easier to use than XMLHttpRequest
or fetch()
.
Here is an example of how to use Axios to make an HTTP GET request:
import axios from 'axios';
axios.get('http://www.example.com/api/data')
.then(response => console.log(response.data))
.catch(error => console.error(error));
In this example, we first import the Axios library.
Then, we use the get()
method to make an HTTP GET request to the specified URL. The then()
method is used to specify a function to be called when the request is successful, and the catch()
method is used to specify a function to be called if the request fails.
In this example, the server's response data is logged to the console on success, and any errors are logged to the console on failure.
You can also pass additional options to the get()
method, such as query parameters or headers. For example:
axios.get('http://www.example.com/api/data', {
params: {
id: 123
},
headers: {
'X-Custom-Header': 'MyValue'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
In this example, we pass an object with params
and headers
properties to the get()
method. The params
property is used to specify query parameters, and the headers
property is used to specify request headers.
You can also use Axios to make other types of HTTP requests, such as POST, PUT, DELETE, etc. by using the appropriate method (e.g. post()
, put()
, delete()
).
The Axios library also provides additional features such as automatic JSON parsing and the ability to create custom HTTP headers.
Best Practices for Making HTTP Requests via JavaScript
What are some of the best practices for making HTTP requests with JavaScript?
- Use
https
instead ofhttp
: Whenever possible, usehttps
instead ofhttp
to make your HTTP requests.https
is more secure thanhttp
because it uses a secure SSL/TLS connection to encrypt the data being transmitted. - Cache your HTTP requests: If you are making requests to the same server or API endpoint multiple times, consider caching the responses to avoid unnecessary network requests. You can use the
Cache
API or a library likeaxios-cache-adapter
to cache HTTP responses. - Use the appropriate HTTP method: Use the appropriate HTTP method for each request based on its intended action. For example, use
GET
for retrieving data,POST
for creating new resources,PUT
for updating existing resources, andDELETE
for deleting resources. - Validate the server's response: Always validate the server's response to ensure that it is in the expected format and contains the expected data. This can help prevent errors and improve the reliability of your application.
- Use error handling: Use error handling to catch and handle any errors that may occur during the request. This can include things like network errors, server errors, and invalid responses.
- Use a polyfill for older browsers: If you need to support older browsers that do not support the
XMLHttpRequest
object or thefetch()
function, you can use a polyfill to provide support for these features. - Use a library: Consider using a library like Axios or Superagent to simplify the process of making HTTP requests. These libraries provide a simpler interface and can make it easier to manage and debug your HTTP requests.
By following these best practices, you can ensure that your HTTP requests are secure, efficient, and reliable. This will help improve the performance and stability of your application.
Considerations when Making HTTP Requests
What are some considerations when making HTTP requests via JavaScript? There are several to keep in mind when making HTTP requests via JavaScript:
- Cross-origin resource sharing (CORS): If you are making HTTP requests to a server or API that is on a different domain than your website, you will need to consider cross-origin resource sharing (CORS). CORS is a security feature that restricts web pages from making requests to a different domain than the one that served the web page. To allow your web page to make requests to a different domain, you will need to enable CORS on the server or API.
- Security: When making HTTP requests, you should consider the security implications of the request. For example, you should avoid sending sensitive data such as passwords or credit card numbers over an unsecured connection. You should also be aware of potential threats such as cross-site scripting (XSS) and cross-site request forgery (CSRF).
- Performance: Consider the performance implications of making HTTP requests. Large or frequent requests can affect the performance of your application, so it is important to optimize your requests and minimize their impact on performance.
- Error handling: It is important to handle errors that may occur during the request, such as network errors or server errors. You should also consider how to handle invalid responses or data from the server.
- Browser support: Different browsers may have different capabilities and limitations when it comes to making HTTP requests. You should consider the browser support for the methods and technologies you are using to make HTTP requests. If necessary, you can use a polyfill to provide support for older browsers.
- Throttling: If your application is making requests too frequently or too rapidly, consider using a rate-limiting or throttling mechanism to reduce the number of requests and their impact on performance. This can help prevent overloading the server and improve the reliability of your application.
- Cache: You should consider caching HTTP responses to reduce the number of requests sent, improve response times and save bandwidth. You can use the Cache API or a library like axios-cache-adapter to cache HTTP responses.
- Compression: Using compression algorithms such as GZIP or Brotli can help reduce the size of responses, which can reduce the amount of data sent and improve response times.
These are just some of the considerations to keep in mind when making HTTP requests via JavaScript. Following best practices will help ensure that you make efficient, secure, and reliable requests that provide a good user experience.
Conclusion
As a web developer, it is essential to understand the implications of making HTTP requests for an optimal user experience when creating applications.
By following best practices and considering various considerations while making HTTP requests in JavaScript, you can ensure your application offers maximum efficiency, reliability and stability. This will provide a more pleasant user experience and greater customer satisfaction.
Additionally, it is important to consider factors such as caching, redirects, timeouts, error handling and other aspects of development that can affect the end result. All these elements are key in creating robust applications that meet user needs with maximum efficiency and reliability.
By taking into account all the necessary components while making HTTP requests in JavaScript, developers can create applications that offer users an enhanced web experience.
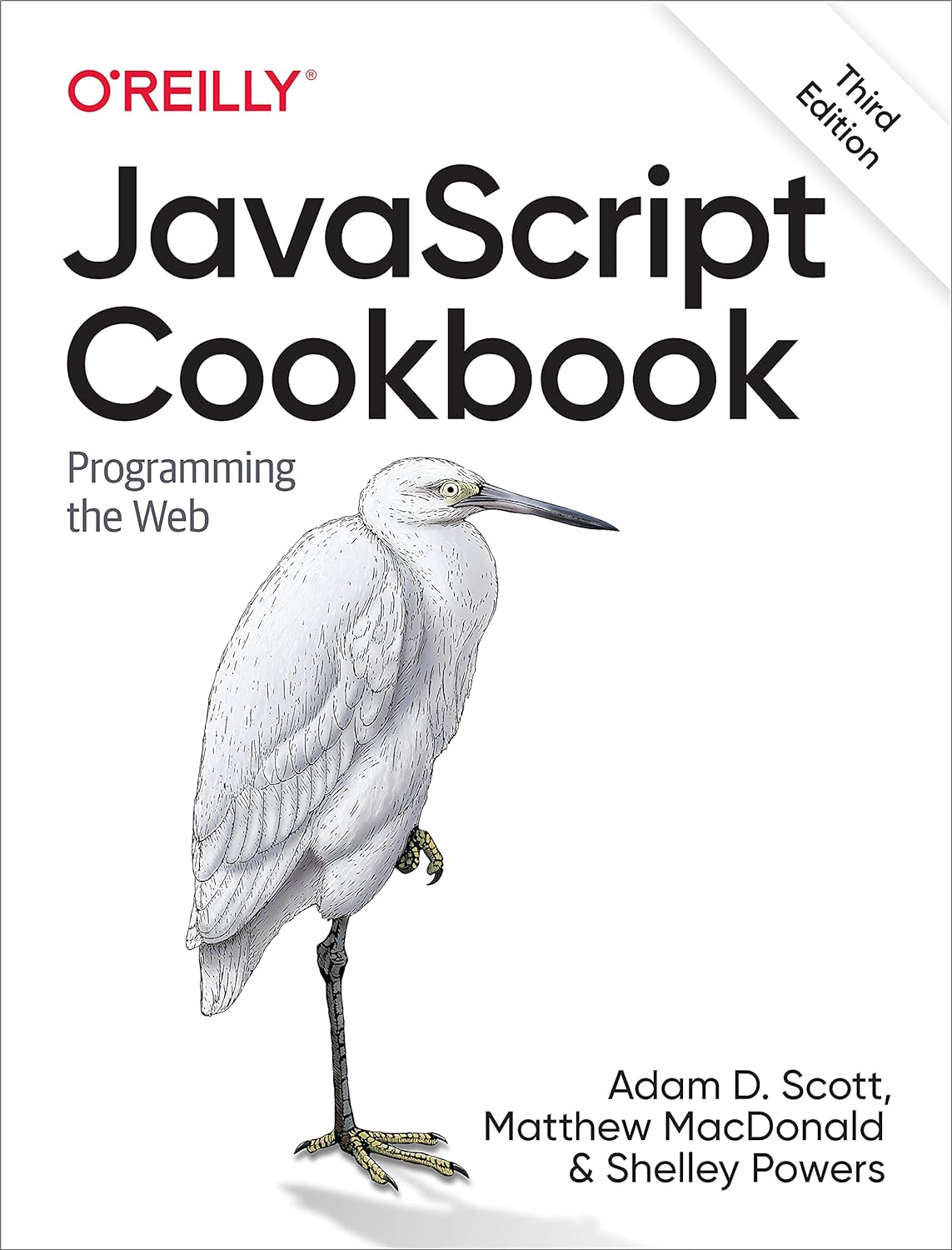
JavaScript Cookbook, 3rd Edition
Why reinvent the wheel every time you run into a problem with JavaScript? This cookbook is chock-full of code recipes for common programming tasks, along with techniques for building apps that work in any browser. You'll get adaptable code samples that you can add to almost any project - and you'll learn more about JavaScript in the process.